Mystery of CORS — Explained Simply
I remember vividly when this question lingered in my mind for the longest time: What exactly is CORS or Cross-Origin Resource Sharing, and why does it even exist?
If you're a web developer or a full-stack developer, chances are you've seen this error before and seen it quite a lot. I've often seen these errors, and hundreds of online articles explain workarounds and how to fix them. But I remember vividly when this question lingered in my mind for the longest time: What exactly is CORS or Cross-Origin Resource Sharing, and why does it even exist?
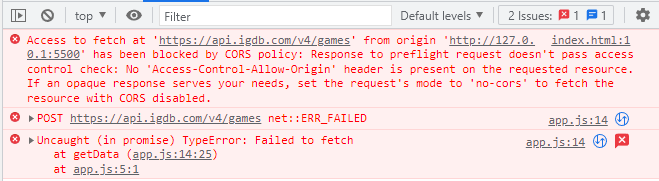
Now, to explain CORS, we need to start with the why. Let's travel through time by following a use case and understanding how it'd play out at different stages before and after the introduction of CORS.
🍪 Cookies and Banking
Let's imagine this scenario: on a fine Sunday morning, you were scrolling through TikTok, and you came across a video of how the latest and greatest iPhone 15 Pro camera outperformed popular Android flagships. You've been saving up for a new phone for the last few months now, and you decided to check your account balance.
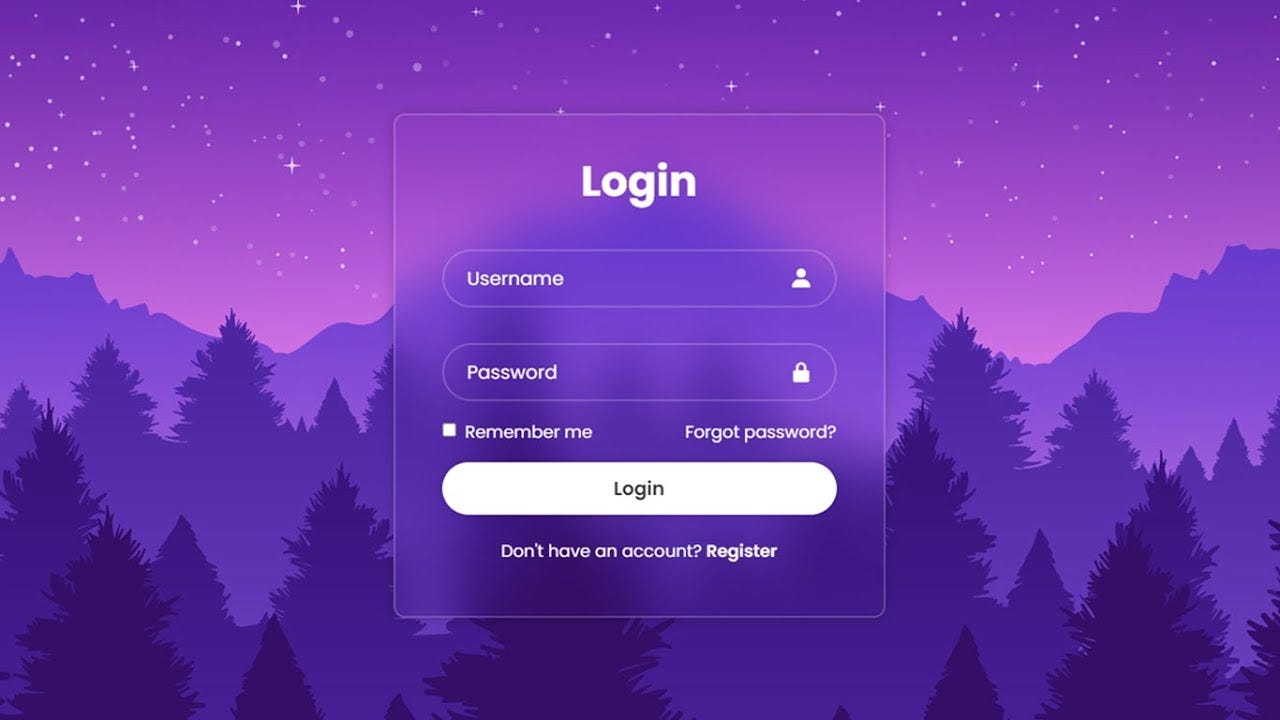
You sign into bank.com
, which is your net banking service. After you've signed in, under the hood, a "session cookie (not a real 🍪)" is stored in your browser.
🙋 Why do we need a session cookie?
A session cookie basically tells the bank servers that your web browser is now logged into your account.
All future requests to bank.com
will now contain this cookie, and the bank servers can now send responses knowing that you're logged in. But wait, beep, beep! You received a notification.
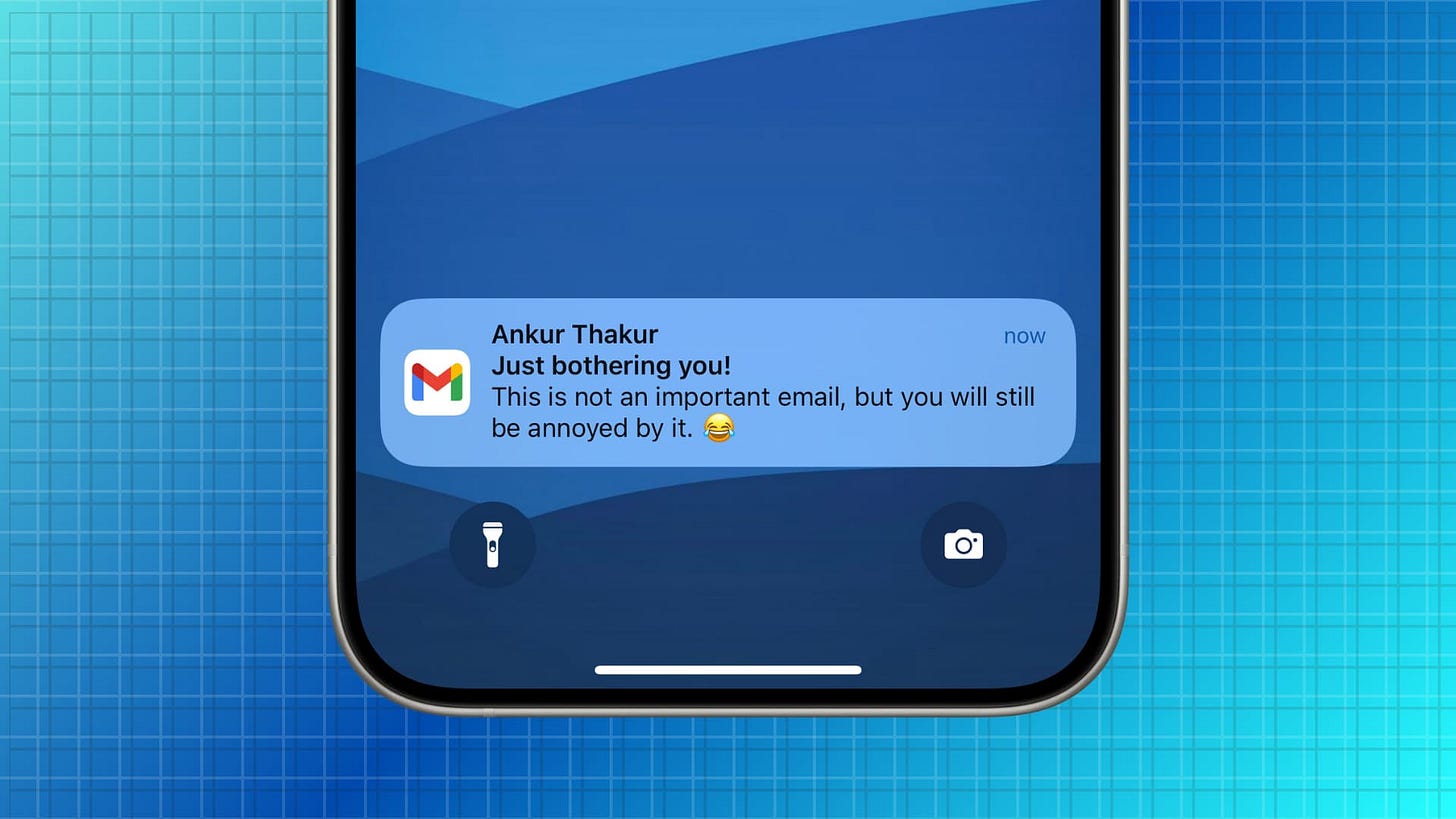
So now, you decided to check your gmail inbox. You see a new email from your friend Ankur and you noticed his email looks different, but hey, maybe he changed it. You see a link containing Amazon discount codes for Apple products. Of course, it's Ankur, and you decide to checkout the link. The link sent you to attack.com
.
Now the following things happen under the hood without your knowledge. This website attack.com
then sends a request to bank.com
to get your banking details. Keep in mind that your bank's internet banking service at bank.com
still thinks that you're logged in and operating because of the session cookie stored in your browser. The bank servers responds with the requested details normally, as for the bank, it just looks like you've requested them. Now the hacker at attack.com
has access to these details and uses them for malicious purposes.
🧼 Advent of Same Origin Policy
Very soon, people realised this was bad, and popular web browsers adopted an SOP (Same-Origin Policy), where if your browser notices that you're trying to make requests to bank.com
servers from anywhere other than bank.com
website, they will be blocked. But, and it's a very important "but", the important thing to note here is that this is a browser based policy. The bank servers really has no way to tell where a request comes from, and hence, it can't protect much against CSRF attacks.
Under the hood...
Your browser will basically step in and check if you're trying to request details for an origin (basically the URL, something like scheme + domain name + port, eg: https://bank.com:4000) that matches your current origin. Only if your current origin and the request origin matches, it will send those requests.
Limitations of SOP
Looks great! But wait, how do I use public APIs with the Same-Origin policy. It wouldn't work at all, unless you use some sort of a proxy solution. In fact, this is one of the biggest limitations of the Same-Origin policy.
Cross-Site Request Forgery (CSRF) Attacks
These attacks forces authenticated users to submit a request to a web application against which they are currently authenticated. Usually executed with the help of social engineering, an attacker may trick the user of the web application into executing malicious actions of the attacker's choosing. You can read more into CSRF Attacks.
Here's the thing: servers can sort of tell where a request came from. Usually requests have an Origin
header, describing the origin of the request. In the above CSRF attack example, the request would look something like this:
The bank servers should, in theory, be checking for this origin
header and make sure it only responds to requests where the origin
makes sense. And it usually does, which makes it more secure and limiting at the same time.
This led to CORS eventually...
🚀 CORS To The Rescue!
If a web application at example.com
attempts to request resources from bank.com
, the browser automatically includes an Origin
header with the value example.com
in the request to indicate where the request originates from. Now, unlike SOP, where cross origin requests are outright blocked by the bank's servers, using it's own CORS policy, the server can inspect this Origin
header and decide whether to allow or deny the request.
I'll make it even simpler to understand using my imperfect metaphor. My wife and I need to step out of the house, and we leave my 10 year old nephew in charge.
Here's Why You Need CORS
We don't give our nephew any instructions on who to let in and what they can do. A random person knocks on the front door, and he lets him in. Then the stranger invites more people, robs our house, spray paints the walls and floor, and steals our car.
CORS gives a framework of who is allowed in and allowed to call whom.
If bank.com
considers example.com
trustworthy, or if the resource it's trying to access is meant for public access, it can respond with specific CORS headers, such as Access-Control-Allow-Origin
, indicating which origins are permitted to access the resource.
If the bank allows example.com
to access the resource, this header might be set to http://example.com
, explicitly allowing this origin, or *
for public resources that any origin can access. The browser is responsible for facilitating all this. If any of it is wrong, you'll get the infamous and nasty CORS error.
Setting the Access-Control-Allow-Origin
We tell our nephew, "Don't allow anyone in except for Mrs Joseph (our neighbour)." Someone knocks on the door, and our nephew asks who is it. He can then decide based on who we told him to allow in.
This header allows you to tell the browser which sites you will allow in.
Setting the Access-Control-Allow-Credentials
We tell our nephew, "Don't allow anyone in except for Mrs Joseph, or anyone who knows our secret code word."
Typically CORS doesn't include cookies or any other authentication in its requests. Setting this header to true throws cookies in the request as well.
Setting the Access-Control-Allow-Methods
We tell our nephew, "You can invite one friend over, but they can't spray paint the wall."
This tells the CORS request to allow what type of requests: GET, PUT, POST, DELETE. If you wanted a site to just fetch data and not update anything, you can use this header.
Setting the Access-Control-Max-Age
We tell our nephew, "You can invite one friend over, but they have to leave at 5PM."
This header sets the value in seconds to cache preflight requests, such as the data in Access-Control-Allow-Headers
and Access-Control-Allow-Methods
headers.
Now what if the request doesn't have the Origin
header? What if it doesn't use one of the basic HTTP methods?
This is where we need to talk about preflight
requests in CORS.
✈️ Preflight Requests
For some types of methods that could potentially modify data on the server (e.g. PUT, DELETE), or requests that use headers that are not automatically included in every request, browsers will first send a preflight request before making an actual request.
💡 Why is a preflight request required?
It is an HTTP OPTIONS request whose primary purpose is to check with the server whether the actual request is safe to send.
The preflight request includes headers that describe the HTTP method and headers of the actual request. The following things happens next:
Server Response
If the server supports the CORS policy and the actual request, it responds to the preflight request with headers like Access-Control-Allow-Methods
and Access-Control-Allow-Headers
indicating what methods and headers are allowed.
Browser Decision
Based on the server's response, the browser decides whether to proceed with the actual request. If the server's response indicates that the request is allowed, the browser sends the actual request and if not allowed, then the browser blocks the request and you'll get the nasty CORS error.
If you've reached here, I hope you understand CORS a bit more in detail. It's all browser policy, and your server must be implemented to comply with it. Thank you for reading, and I hope this was useful for you.
Have a wonderful day!
Sanjay.
P.S. If you'd like to support my writing, you can:
Or